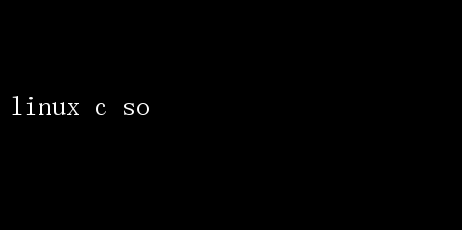
Linux C Shared Objects: Unleashing the Power of Dynamic Linking
In the realm of software development, the ability to create modular, reusable, and efficient code is paramount. Linux, with its robust operating system architecture and extensive developer community, offers a multitude of tools and techniques to achieve this. Among these, the concept of C Shared Objects(SOs), also known as Shared Libraries, stands out as a cornerstone for dynamic linking and code reuse. This article delves into the intricacies of C SOs on Linux, highlighting their significance, implementation, benefits, and advanced usage scenarios.
Understanding Shared Objects
Shared Objects(SOs) are special types of files in Unix-like operating systems, including Linux, that contain executable code and data which can be used by multiple programs simultaneously. They are akin to static libraries but with a critical difference: while static libraries are linked into the executable at compile time, making the executable larger and potentially redundant if multiple programs use the same library, shared libraries are linked at runtime. This dynamic linking mechanism allows for smaller executable sizes, memory conservation, and easier updates to library code without requiring recompilation of dependent programs.
In the context of C programming, shared libraries typically have the`.so` extension, signifying shared object. They are created using the GNU CompilerCollection (GCC) or other compatible compilers with specific flags and tools.
Creating Shared Objects
Creating a shared object in Linux involves several steps, starting from writing the source code to compiling and linking it intoa `.so` file. Here’s a simple example to illustrate the process:
1.Writing the Source Code:
Suppose we have a simple math library with two functions:`add` and`subtract`.
c
//math_functions.h
ifndef MATH_FUNCTIONS_H
define MATH_FUNCTIONS_H
intadd(int a, intb);
intsubtract(int a, intb);
endif //MATH_FUNCTIONS_H
c
//math_functions.c
include math_functions.h
intadd(int a, intb){
return a + b;
}
intsubtract(int a, intb){
return a - b;
}
2.Compiling and Linking:
To create a shared objectfrom `math_functions.c`, we usethe `-fPIC` (Position IndependentCode) flag during compilation andthe `-shared` flag during linking.
bash
gcc -fPIC -c math_functions.c -omath_functions.o
gcc -shared -o libmath.somath_functions.o
Thisproduces `libmath.so`, our shared library.
Using Shared Objects
Once a shared object is created, it can be used by other programs. Here’s how you would link and run a program that uses`libmath.so`:
1.Writing the Main Program:
c
// main.c
include
include math_functions.h
intmain(){
int a = 5, b = 3;
printf(Add: %d + %d = %dn, a, b,add(a,b));
printf(Subtract: %d - %d = %dn, a, b,subtract(a,b));
return 0;
}
2.Compiling and Linking the Main Program:
When compiling the main program, we need to specify the shared library during linking using the`-L` flag to indicate the library path andthe `-l` flag to specify the library name(withoutthe `lib` prefix and`.so` suffix).
bash
gcc -o main main.c -L. -lmath
3.Running the Program:
Before running the program, we need to ensure that the shared library is found at runtime. This can be done by setting the`LD_LIBRARY_PATH` environment variable to include the directory containing`libmath.so`.
bash
exportLD_LIBRARY_PATH=.:$LD_LIBRARY_PATH
./main
This should output:
Add: 5 + 3 = 8
Subtract: 5 - 3 = 2
Benefits of Using Shared Objects
The adoption of shared objects in Linux C programming yields several significant benefits:
- Memory Efficiency: Shared libraries are loaded into memory once and shared among all processes that use them, reducing overall memory usage.
- Disk Space Savings: Since multiple programs can share the same library file, disk space is conserved.
- Ease of Updates: Updating a shared library does not require recompilation of dependent programs, facilitating easier maintenance and security patches.
- Code Reusability: Encourages modular design, where commonly used functionalities are encapsulated in libraries, promoting code reuse and reducing redundancy.
- Performance: Dynamic linking can sometimes improve load times because only necessary parts of the library are loaded into memory when needed.
Advanced Usage and Considerations
While the basics of creating and using shared objects are straightforward, there are several advanced considerations and techniques worth exploring:
- Versioning: Handling multiple versions of a shared library can be complex. Linux provides mechanisms suchas `soname` (Shared ObjectName) and symbolic links to manage versions efficiently.
- Dependency Management: Toolslike `ldconfig` help manage shared library dependencies by maintaining a cache of available libraries and their locations.
- Symbol Visibility: Controlling whichsymbols (functions andvariables) are exported from a shared library can improve security and reduce the librarys attack surface.The `__attribute__((visibility(default)))` GCC attribute can be used for this purpose.
- Thread Safety: When developing thread-aware applications, ensuring that shared libraries are thread-safe is crucial. This often involves using thread-local storage and proper synchronization primitives.
- Debugging and Profiling: Debugging shared libraries can be challenging due to their dynamic nature. Tools like`gdb`